Pre-signed URLs to upload/download files
Sirv provides multiple ways to protect your files, including JWT signed URLs with Sirv's REST API or pre-signed URLs using the Sirv S3 API. This page describes how to use pre-signed URLs.
Pre-signed URLs are special URLs that give access to a file for a temporary period to anyone you share the URL with. These URLs can be embedded in a web page or used in other ways to allow secure download or upload files to your Sirv account, without sharing your S3 login credentials.
Pre-signed URLs are easily created on the Sirv backend, containing a signature for validation and optionally an expiry time. These 'signature' and 'expires' fields validate the URL on the Sirv platform, so that you're certain the URL is trusted.
Creating a pre-signed URL
You can generate pre-signed URLs using any of the AWS SDKs.
This code snippet uses the AWS SDK for JavaScript to generate a URL with no expiry, using your Sirv S3 keys:
var AWS = require('aws-sdk'); AWS.config.update({ accessKeyId: 'ENTER_YOUR_SIRV_S3_ACCESS_KEY_HERE', secretAccessKey: 'ENTER_YOUR_SIRV_S3_SECRET_KEY_HERE' }); var s3 = new AWS.S3({ endpoint: new AWS.Endpoint('https://s3.sirv.com'), s3ForcePathStyle: true }); s3.getSignedUrl('putObject', { Bucket: 'ENTER_YOUR_SIRV_S3_BUCKET_HERE', Key: 'example.jpg' }, function (err, url) { console.log(err, url); });
Note how the 's3ForcePathStyle: true' option is used to prevent an SSL certificate error.
The following code snippet adds an expiry time of 60 seconds:
var AWS = require('aws-sdk'); AWS.config.update({ accessKeyId: 'ENTER_YOUR_SIRV_S3_ACCESS_KEY_HERE', secretAccessKey: 'ENTER_YOUR_SIRV_S3_SECRET_KEY_HERE' }); var s3 = new AWS.S3({ endpoint: new AWS.Endpoint('https://s3.sirv.com'), s3ForcePathStyle: true }); var params = { Bucket: 'bucket', Key: 'key', Expires: 60 }; var url = s3.getSignedUrl('getObject', params); console.log('The URL is', url); // expires in 60 seconds }; s3.getSignedUrl('putObject', { Bucket: 'ENTER_YOUR_SIRV_S3_BUCKET_HERE', Key: 'example.jpg' }, function (err, url) { console.log(err, url); });
Uploading a file
Once a URL has been generated, you can use it to upload a file with a utility such as curl:
curl -v -T ./DSC_5410.JPG 'https://s3.sirv.com/yoursirvaccount?AWSAccessKeyId=abcdefghij1234567890&Expires=123456789&Signature=zxywvutsr0987654321'
The screenshot below shows how it looks when a pre-signed URL is generated and then a local file is uploaded (located at ~/Downloads/DSC_5410.JPG) with the curl utility:
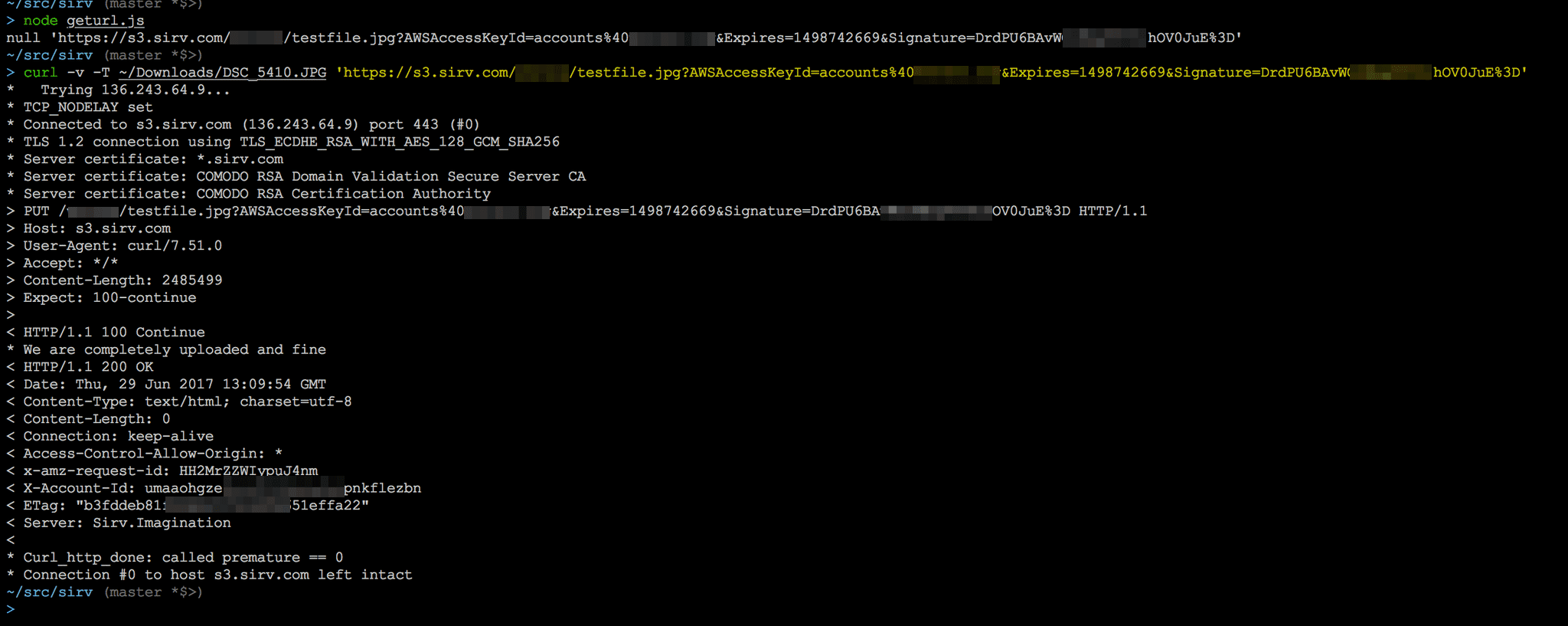
Additional parameters
Refer to the official AWS SDKs for additional parameters to your URLs: